Python for Beginners
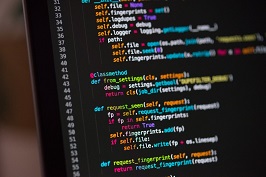
General Information
- This course is an online introductory program.
- Learning environment will be through an online conferencing tool.
- This course duration is 12 hours. Each class 90 minutes.
- Course will be held on Thursday at 6:00pm EST.
- Minimum 5 participants to run one class.
- At the end of the program, each participant will receive an attendance certificate
Prerequisites
- Fair knowledge of English Speaking.
- A PC and internet connection
- No previous programming knowledge is requiired.
Course Description
Python is one of the fastest growing programming languages. It allows you to write programs in fewer lines of code than many other languages. It is also used in scientific fields for academic research and applied work. This course will put your feet on the first steps on how to start coding using Python. You will learn the basic syntax of how the Python language works.
Course Objective
By the end of this course, student should be able to:
- Learn Python Basics to gain the confidence to build larger and more complex program
- Learn to use Functions, Lists, Tuples and Sets
- Understand flow control using conditions
- Use loops
-
1IntroductionAn IDE (or Integrated Development Environment) is a program dedicated to software development. As the name implies, IDEs integrate several tools specifically designed for software development. These tools usually include: - An editor designed to handle code (with, for example, syntax highlighting and auto-completion) - Build, execution, and debugging tools - Some form of source control
-
2Basic Python syntaxYou will start learning the structure of a python program and how to run it through simple Print statements. These simple programs will make you familiar with the coding environment and enhancing your code incrementally.
-
3Strings and console outputIn this lesson, you will learn about different data types you can use in Python. A variable is a container for a value. It can be assigned a name, you can use it to refer to it later in the program.
-
4Working with strings and numbersA string in Python is a sequence of characters. It is a derived data type. In this class, you will learn to create, format, modify and delete strings in Python. Also, you will be introduced to various string operations and functions.
-
5Conditional and flow controlConditional statement is a statement that controls the flow of execution depending on some condition. In Python the keywords if, elif, and else are used for conditional statements.
-
6FunctionsLoops are important in Python or in any other programming language as they help you to execute a block of code repeatedly. In this class, you will learn about the "while" and "for" loops.
-
7Lists and dictionariesLists are used to store multiple items in a single variable. In this class, you will learn how lists are created, adding or removing elements, and more.
-
8LoopsTuples are sequences, just like lists. The differences between tuples and lists are, the tuples cannot be changed unlike lists and tuples use parentheses, whereas lists use square brackets. In this class, you will learn what tuples are, how to create them, when to use them, what operations you perform on them.
-
9Introduction to classesA Function in Python is a piece of code which runs when it is referenced. It is used to utilize the code in more than one place in a program. It is also called method or procedure. Python provides many inbuilt functions and it allows you to define your own functions. In this class, you will learn how to create user-defined functions and how to pass parameters to them.
-
10File input and output
Be the first to add a review.
Please, login to leave a review